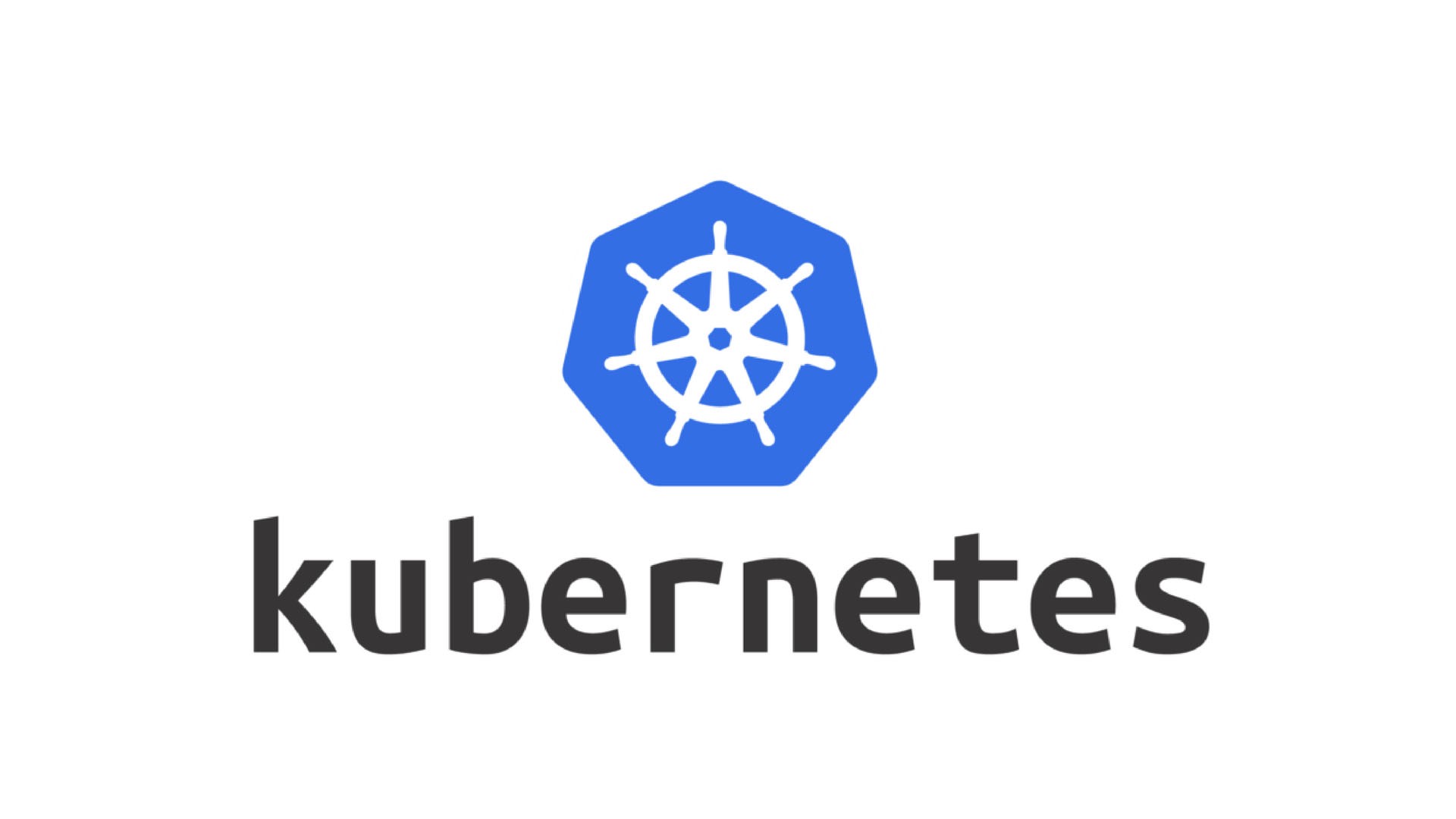
Kubectl Useful Commands
July 21, 2021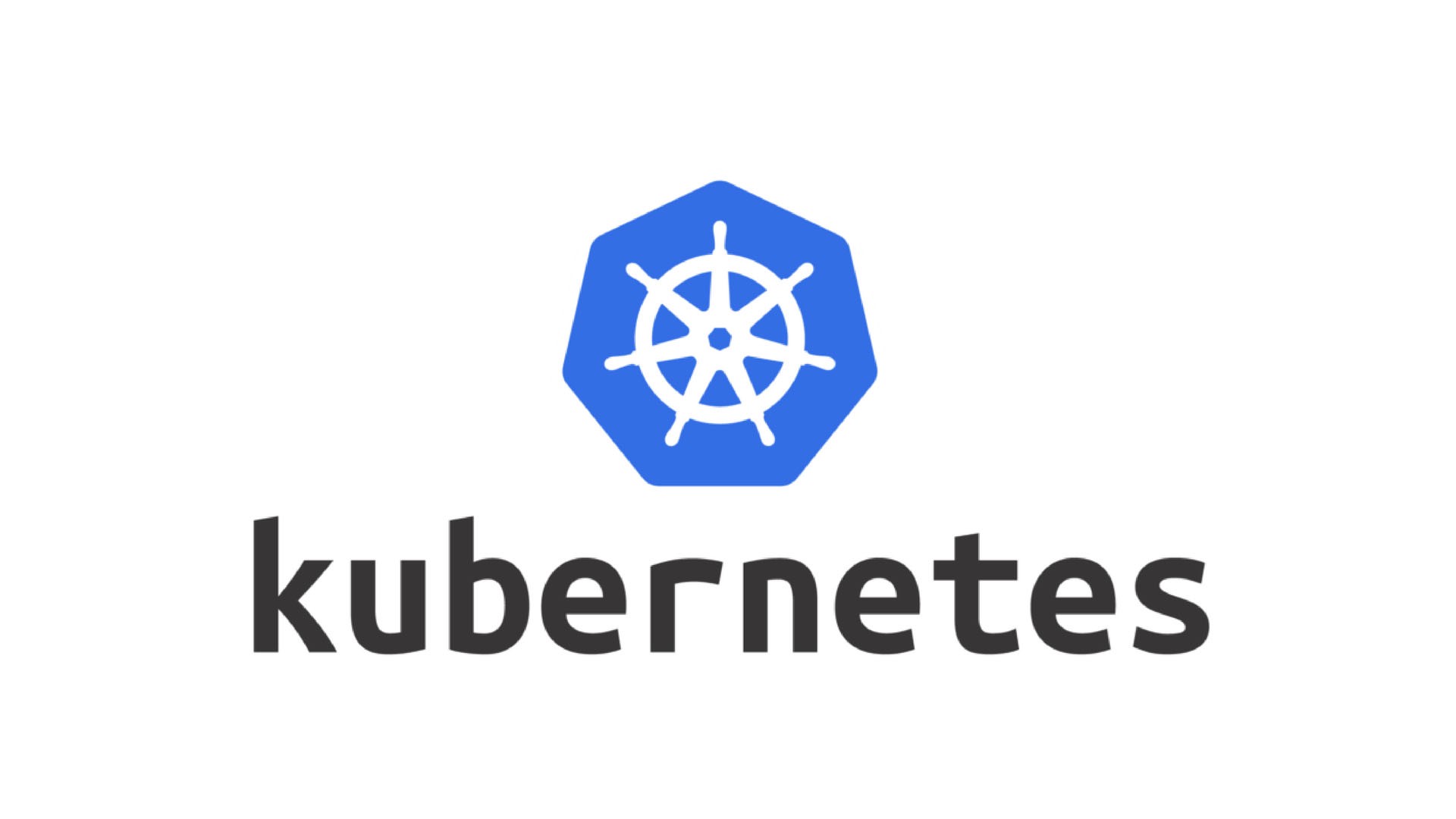
po : Pods
rs : ReplicaSets
deploy : Deployments
svc : Services
ns : Namespaces
netpol : Network policies
pv : Persistent Volumes
pvc : PersistentVolumeClaims
in : Service Accounts
Backup
kubectl get all -A -o yaml > backup.yaml
Explain
kubectl explain sc –recursive | less
Pods
#List Pod
kubectl get pods
kubectl get pods -o wide
kubectl get pods -n kube-system
kubectl get pods –selector app=test-application,env=develop
kubectl get pods -l app=test-application,env=develop
kubectl get pods –all-namespaces
kubectl get pods –show-labels
#Pod Status
kubectl describe pod mypod
#Create Pod
kubectl run mypod –image nginx
#Edit Pod
kubectl edit pod mypod
kubectl get pod mypod -o yaml > mypod.yaml
#Create Pod from YML file
kubectl create -f mypod.yml
kubectl apply -f mypod.yml
#Delete Pod
kubectl delete pod mypod
ReplicaSet
#Create ReplicaSet
wget https://kubernetes.io/examples/controllers/frontend.yaml
cat frontend.yaml
apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: frontend
labels:
app: guestbook
tier: frontend
spec:
# modify replicas according to your case
replicas: 3
selector:
matchLabels:
tier: frontend
template:
metadata:
labels:
tier: frontend
spec:
containers:
– name: php-redis
image: gcr.io/google_samples/gb-frontend:v3
kubectl apply -f https://kubernetes.io/examples/controllers/frontend.yaml
#Get ReplicaSet
kubectl get rs
#Delete ReplicaSet
kubectl delete rs frontend
Deployment
#Scale Deployment
kubectl replace -f application.yml
kubectl scale –replicas=10 -f application.yml
kubectl scale –replicas=10 replicaset application
#Generate YML File From Deployment
kubectl create deployment –image=nginx nginx –replicas=2 –dry-run=client -o yaml > nginx.yaml
kubectl create deployment httpd-name –image=httpd
kubectl scale deployment httpd-name –replicas=10
#Rollout
kubectl rollout status deployment/httpd-name
kubectl rollout history deployment/httpd-name –revision=1
kubectl rollout undo deployment/httpd-name
#Edit deployment on environment
kubectl edit deploy my-service-name dev
Configuration Examples
– Pod Environment variable
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
env:
– name: DB_NAME
value: MyDB
– name: DB_URL
valueFrom:
configMapKeyRef:
name: config-url
key: db_url
– name: DB_PASSWORD
valueFrom:
secretKeyRef:
name: config-passwd
key: db_password
– ConfigMap
#Create ConfigMap
kubectl create configmap testconfigmap –from-literal=TestKey1=TestValue1 –from-literal=TestKey2=TestValue2
kubectl create configmap testconfigmap –from-file=/opt/test_file
#Test
kubectl get configmaps
kubectl describe configmaps
kubectl describe configmap testconfigmap
– Use CofigMap in Pod
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
envFrom:
– configMapRef:
name: testconfigmap
– Secrets
#Create Secrets
kubectl create secret generic testsecret –from-literal=Key1=Value1 –from-literal=Key2=Value2
kubectl create secret generic testsecret –from-file=/opt/secret
#Test
kubectl get secrets
kubectl describe secrets
kubectl get secret testsecret
kubectl describe secret testsecret
kubectl get secret testsecret -o wide
– Use Secret in Pod
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
envFrom:
– secretRef:
name: testsecret
– Security Context
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
securityContext:
runAsUser: 1000
capabilities:
add: [“ADMINISTRATOR”]
containers:
– name: nginx
image: nginx
command: [“printenv”]
args: args: [“HOSTNAME”]
securityContext:
runAsUser: 2000
capabilities:
add: [“USER”]
– Service Account
#Create Service Account
kubectl create serviceaccount testsa
– Use Service Account in Pod
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
serviceAccount: testsa
containers:
– name: nginx
image: nginx
envFrom:
– secretRef:
name: testsecret
– Resource requirements
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
resources:
requests:
memory: “1Mi”
cpu: 0.2
limits:
memory: “1Gi”
cpu: 1
envFrom:
– secretRef:
name: testsecret
– Taints Node: NoSchedule , PreferNoSchedule , NoExecute
#Create Taints
kubectl taint nodes vagrant example-key=blue:NoSchedule
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
envFrom:
– secretRef:
name: testsecret
tolerations:
– key: “example-key”
operator: “Equal”
value: “blue”
effect: “NoSchedule”
#Remove
kubectl taint nodes vagrant example-key=blue:NoSchedule-
– Node Selector
#Create Selector
kubectl label nodes vagrant label-key=label-name
– Use in pod.yml
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
envFrom:
– secretRef:
name: testsecret
nodeSelector:
label-key: label-name
Services
kubectl expose deployment testdeployment –name=nginx-service –type=NodePort –target-port=8080 –port=80
kubectl expose pod mypod –port=80 –name=nginx-service –type=NodePort
kubectl create service nodeport mypod –tcp=80:80 –node-port=30080
Namespace
#Get Pods
kubectl get pods –namespace=develop
kubectl get pods -n develop
kubectl get pods –all-namespaces
kubectl get ns
#Change Default Namespace
kubectl config set-context –current –namespace=develop
Readinesss Probe / Liveness Probe
#HTTP Test
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
readinessProbe/livenessProbe:
httpGet:
path: /health
port: 80
initialDelaySeconds: 10
periodSeconds: 5
failureThreshold: 8
envFrom:
– secretRef:
name: testsecret
#TCP Test
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
readinessProbe/livenessProbe:
tcpSocket:
port: 80
envFrom:
– secretRef:
name: testsecret
#Run Command
apiVersion: v1
kind: Pod
metadata:
name: static-web
labels:
role: myrole
spec:
containers:
– name: nginx
image: nginx
readinessProbe/livenessProbe:
exec:
command:
– cat
– probe.htm
envFrom:
– secretRef:
name: testsecret
Logs
kubectl logs -f pod-name
Jobs
#Create Jobs
kubectl create job test-job –image=nginx
#Get Jobs
kubectl get jobs test-job
kubectl get jobs
— Updating —
#ntechdevelopers
[…] + https://blog.ntechdevelopers.com/kubectl-useful-commands/ – Serverless […]